Javascript Promise
What is Promises
A promise is an object that may produce a single value some time in the future: either a resolved value, or a reason that it’s not resolved (e.g., a network error occurred). A promise may be in one of 3 possible states: fulfilled, rejected, or pending. Promise users can attach callbacks to handle the fulfilled value or the reason for rejection.
Promises are eager, meaning that a promise will start doing whatever task you give it as soon as the promise constructor is invoked.
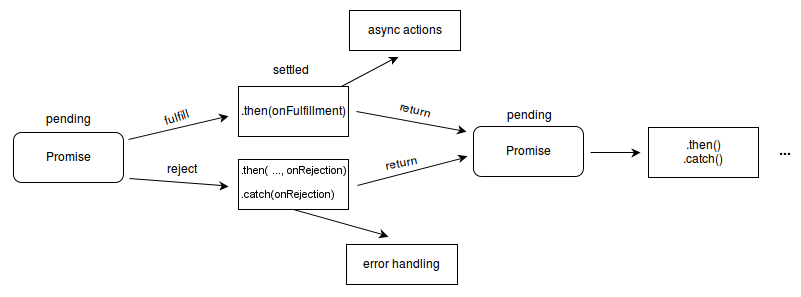
State of Promises
- Pending, when the final value is not available yet. This is the only state that may transition to one of the other two states.
- Fulfilled, when and if the final value becomes available. A fulfillment value becomes permanently associated with the promise. This may be any value, including
undefined
. - Rejected, if an error prevented the final value from being determined. A rejection reason becomes permanently associated with the promise. This may be any value, including
undefined
, though it is generally anError
object, like in exception handling.
Let's look example of Promise
const fetchUser = () => {
return new Promise((resolve, reject) => {
setText('Fetching User...');
setTimeout(() => {
resolve({ name: "Navnit" });
}, 2000);
});
};
fetchUser().then((response)=> console.log(response)); // Promise Resolved with { name: "Navnit" }
// We can write in other way by passing console.log function directly to then
fetchUser().then((response)=> console.log(response)) // Usefull while debugging
Handle Promise
There is two way to handle promise in JavaScript by using then and catch function and by using async await.
We show then and catch in above example let's create example of async await
const url = "https://jsonplaceholder.typicode.com/posts/1";
const waitTillEnd = async url => {
try {
const response = await fetch(url);
const json = await response.json();
console.log(json);
} catch (error) {
console.log(error);
}
};
waitTillEnd(url);
Promise.all()
The Promise.all()
method returns a single Promise
that fulfills when all of the promises passed as an iterable have been fulfilled or when the iterable contains no promises. It rejects with the reason of the first promise that rejects.
It is typically used after having started multiple asynchronous tasks to run concurrently and having created promises for their results, so that one can wait for all the tasks being finished.
const promise1 = Promise.resolve(3);
const promise2 = 42;
const promise3 = new Promise(function(resolve, reject) {
setTimeout(resolve, 100, 'foo');
});
Promise.all([promise1, promise2, promise3]).then(function(values) {
console.log(values);
}); // expected output: Array [3, 42, "foo"]