How to create and publish a node_module on npm registry for any JavaScript Project (NPM Introduction)
In this blog we will go through the fundamentals of node_modules and we will also clear the foundation of it!
Overview
- Definition
- How to create a npm account
- Type of npm packages
- Types of modules
- Install node.js and npm globally in your machine
- How to create a node_module
- What is a package.json file?
- Publish your first node_module on npm registry
- Use your package in your projects
- Find difference between previous and current version
- Quick introduction with Github + NPM
Let's begin!!
Definition: What is npm?
npm is a package manager for the JavaScript programming language. It is the default package manager for the JavaScript runtime environment Node.js. It consists of a command line client, also called npm, and an online database of public, paid-for private packages and scope packages, called the npm registry.
How to create a npm account?

There are two ways to create a npm account:
- Official Website
Creating a npm account is easy from website just to this below link
https://www.npmjs.com/signup
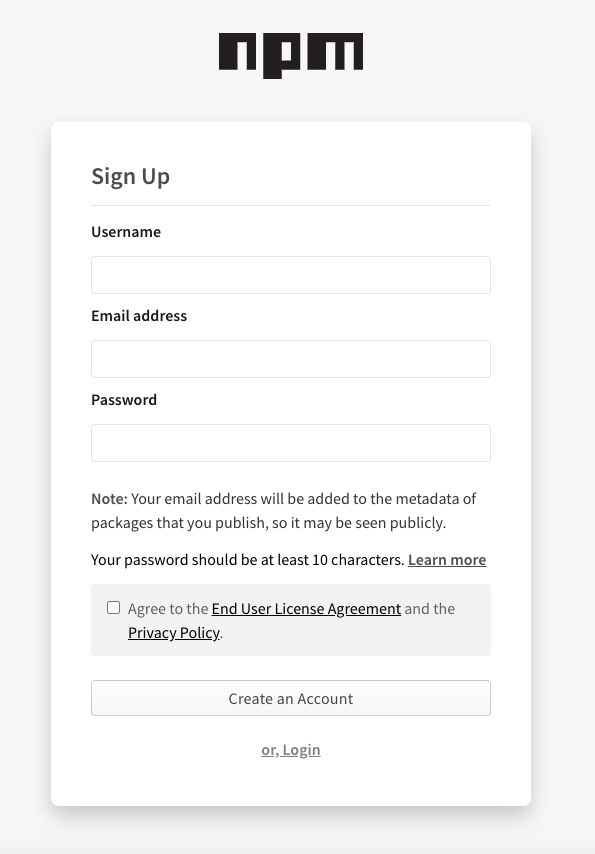
- Command line interface:
npm adduser
Execute this command in your terminal, then simply it will ask for you to enter username, password, etc. And this way you account has now been created on npm registryOnce done you can check if your user is created successfully by visiting npmjs.org/~yourusername
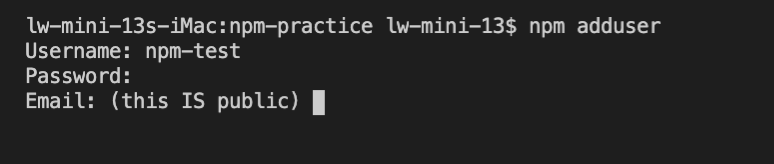
Type of npm packages
There are two types of packages; Public and Private
- Public
There are easily accessible to anyone in this whole wide world with documentation all such things - Private
These are only accessible within the organisation only. If you are out of organisation then you need to ask for their permission. But anyways these private packages will not be visible publicly unless you get permission
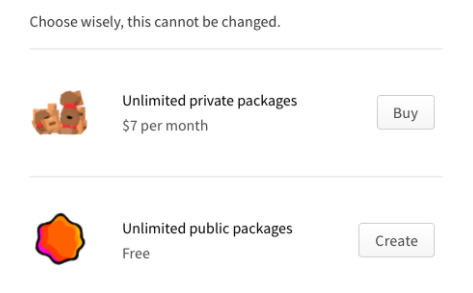
(Note: The above image has pricing displayed which maybe change by npm)
Types of modules
There are two types of modules; scoped and unscoped
- @scoped
Any package which starts from ‘@’ symbol then you can simply consider it as a scoped node module. The below image shows the clear example of a scoped module. As you can see you can deploy as many as modules under single scope so the benefit is that your already congested node_modules folder will not become more messy ;)
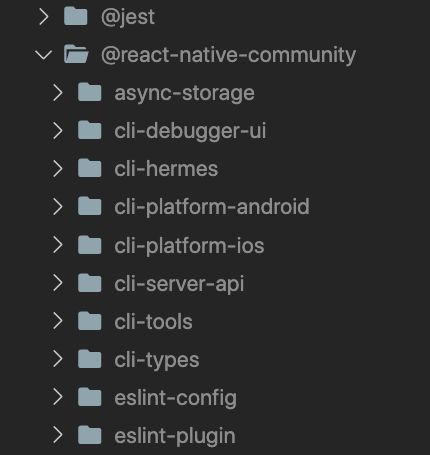
- unscoped
It's the vice-a-versa of the scoped package. See below example
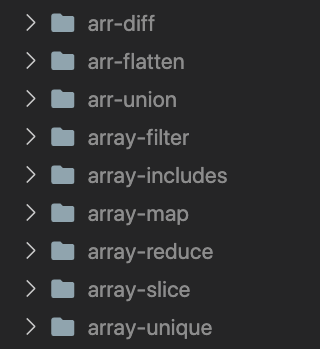
Install node.js and npm globally in your machine
Install latest node.js from the official website
https://nodejs.org/en/
If installed already then make sure it is up-to-date (optional part)
When done you can now use npm
command globally on your machine!
Install npm globally
npm install npm@latest -g --save-exact
-g : It will tell npm command to install/update a package globally on your machine
@latest : It will tell npm command to install the latest npm package
@1.0.x : It will tell npm command to install specific version
-exact: It will tell npm command to install the exact version of the package
If you don't mention -exact option in your command then it will install a package something like this
"esb-helpers": "~1.0.0"
It will be installed with equivalent sign. It means that it will take the latest version of that package series which may or may not be compatible when you have initially installed during the project development. So if you mention -exact then it is a good advantage for you project.
"esb-helpers": "1.0.2"
You can also set it globally if you want to:
npm config set save-exact true
How to create a node_module
Creating a node module is very easy. If you create any project using npm and you can deploy the same as node module. That’s it you are done, LOL ;)
Let's begin for real!
Let’s take an example of a React projects
In most React projects, developers create a components folder where there are custom defined UI components. There are custom views, custom text, custom button, etc but still we create a separate component folder to keep the app design consistent throughout the development.
And we also create a separate utils folder where we define our common functionalities which will be used throughout the application
So considering the above two scenarios we can use those and deploy those as node package i.e; node_module
Here we will try to create a simple node module.
Note: Below example is based on the Linux and MacOS
Step 1: Create a folder example; esb-helpers
Step 2: npm init
Execute this command in your terminal then it will ask for some information like package name, version number, author, etc
This will create a package.json file
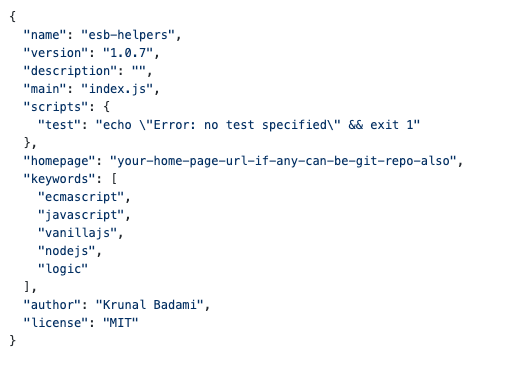
Step 3: touch index.js
This will create an index.js file
You can now code anything you want here
Example of my index.js file:
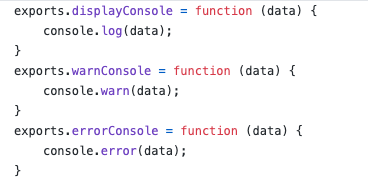
Step 4: Apply proper formatting
Step 5: Add comments to your code
Step 6: Add comments to the exportable functionality so that intelli sense will let other developers know what the functionality is about
Example:
/**
* Fires when a user has finished scrolling.
*/

Step 7: Do testing of your code
Step 8: Update your readme
Step 9: Package done!
What is a package.json file?
All npm based javascript projects contain a file, usually in the project root, called package. json - this file holds various metadata relevant to the project. This file is used to give information to npm that allows it to identify the project as well as handle the project's dependencies
In short way, the folder which contains the pacakge.json file can be knows as Javascript project and node_module as well
There are many types of dependencies:
dependencies
These are your normal dependencies, or rather ones that you need when running your code (e.g. React or ImmutableJS).
devDependencies
These are your development dependencies. Dependencies that you need at some point in the development workflow but not while running your code (e.g. Babel or Flow).
peerDependencies
Peer dependencies are a special type of dependency that would only ever come up if you were publishing your own package.
Having a peer dependency means that your package needs a dependency that is the same exact dependency as the person installing your package. This is useful for packages like react
that need to have a single copy of react-dom
that is also used by the person installing it.
https://classic.yarnpkg.com/en/docs/dependency-types/
https://docs.npmjs.com/cli/v6/configuring-npm/package-json
Publishing a node_module on npm registry
Step 1: npm login
You have to enter your username and password here which you entered during signup
Step 2: Upgrade your version in package.json (only when publishing a new release)
Whenever you publish your node_module you need to mention a version number which stats that this version had these much functionalities
And whenever you update your node_module you need to increase you version number which will let user know that new version has been released with new updates

Step 3: npm publish
You will get a success message in the terminal with a plus symbol which will state that your package has been publish successfully
Step 4: Done!
You can now go to your npm profile and your newly publish npm package will be visible
Note: Sometimes it takes a minute to update in your profile. So don't worry!
If you get some errors, the check if you are logged in or not and also check your current working directory.
If you get errors while updating your package then make sure about version number increment,
Use your package in your projects
Now you can simple install your node_module
Using below commandnpm install esb-helpers@latest --save-exact
Now in your project files you can simply import your node_module and use it!
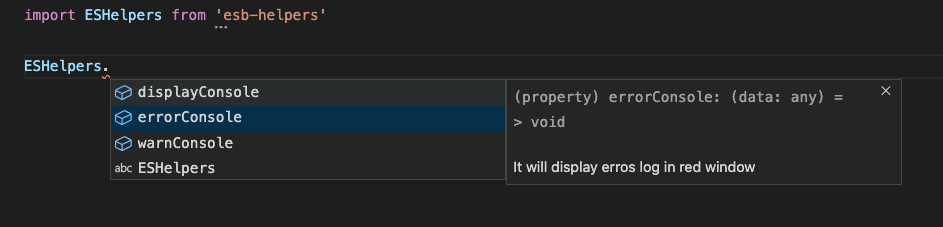
As you can see in the above image, we have imported a our custom node_module
You can see that there are three functionalities available in that package which is displayed in the intelli sense list. If you had added comments in your node_module then it will display something like above
Find difference between previous and current version
If you want to find a difference between of a node_module then you can use this link.
https://diff.intrinsic.com/
This website was developed by Intrinsic Security ( Under VMware )
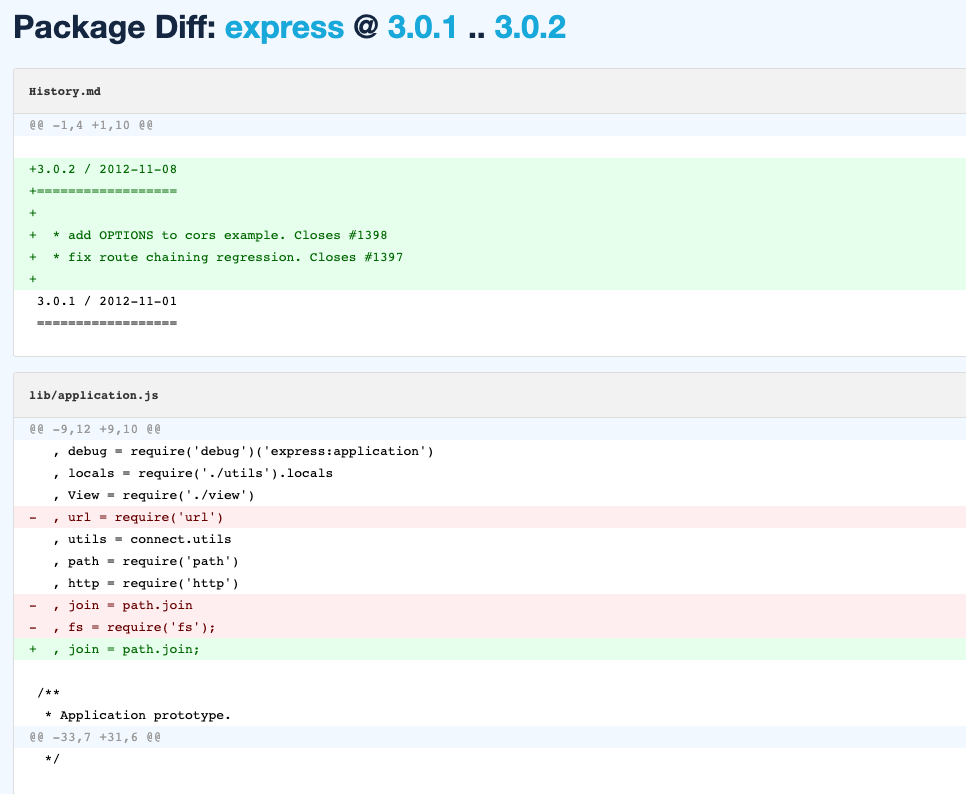
Quick introduction with Github + NPM
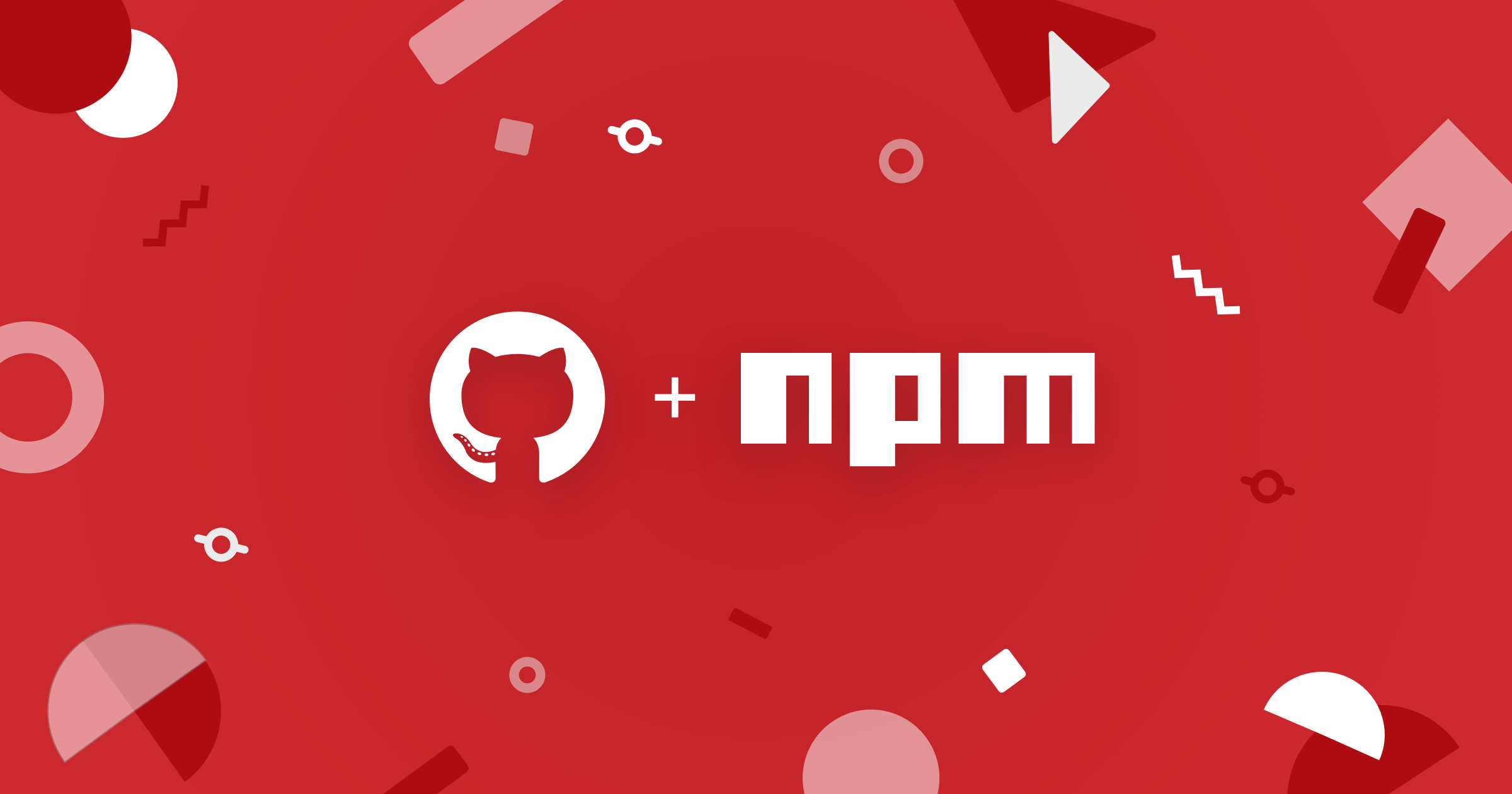
npm is officially part of GitHub now!
Now publishing your package on official npm registry we have learned
But we can also publish our packages on Github, Gitlab, etc has well
Let's dig more into this real quick!!!
Whatever you have done for the current package it’s okay. Worry not there is no extra configuration for this thing you just need to add few lines in your package.json and you're done!
"publishConfig": {
"registry": "https://npm.pkg.github.com/"
},
"repository": {
"type": "git",
"url": "your-git-link",
"directory": "esb-helpers"
},
Use this command to login into Github NPM registrynpm login --registry=https://npm.pkg.github.com/
Here it will ask for a password.
This password will be your access token not your Github password.
You can generate your access token from Github Developer settings
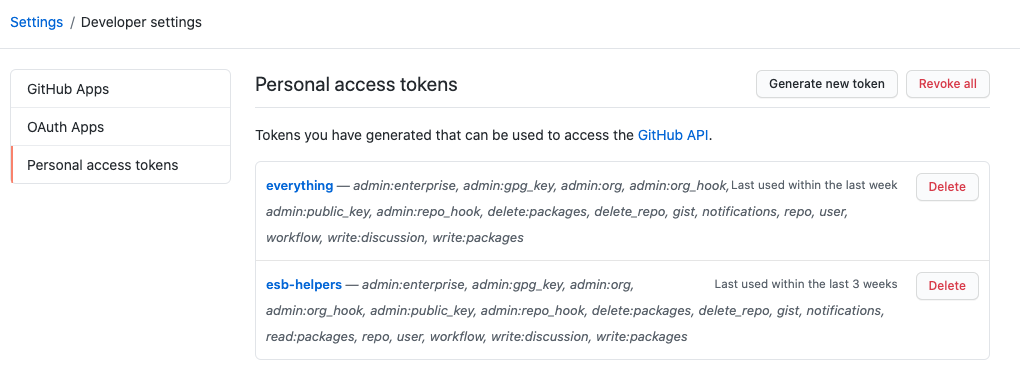
And also there's one downside here.
In the npm registry you were able to publish packages with any comfort name.
But here in Github npm there are scope packages compulsory.
So for normal npm if package name is esb-helpers
then for Github npm it will be @username/esb-helpers
Example:"name": “esb-helpers
- Normal NPM package"name": “@username/esb-helpers”
- Github NPM package
So if you have install a scope package then your command will look somethings like thisnpm install @username/esb-helpers
That’s all folks! </>
Thank you very much for reading and happy coding!