HandleBar.js
What is handlebars ?
Handlebars is a simple templating language. It uses a template and an input object to generate HTML or other text formats. Handlebars templates look like regular text with embedded Handlebars expressions.
A handlebars expression is a {{
, some contents, followed by a }}
. When the template is executed, these expressions are replaced with values from an input object. let’s dive into how to use handlebars.
To install handlebar use following command
npm install handlebars
or
yarn add handlebars
handlebars file use extension .handlebars and you can use that template in any .js file by importing template. There are just a few steps necessary to get things up and running with Handlebars. First, you must create your template in the HTML file.
The next step is to write the necessary JavaScript. This code will contain references to the template along with the context object which is the source of the data being passed to the template.

Let's build template using user data
{
users : [
{ name: "Yehuda", email: "Katz" },
{ name: "Carl", email: "Lerche" },
{ name: "Alan", email: "Johnson" }
]
}
<head>
</head>
<body>
<div>
{{#if users.length}}
{{#each users}}
<table>
<tbody>
<tr>
<td>User Name: {{this.name}}</td>
</tr>
<tr>
<td>User Email: {{this.email}}</td>
</tr>
</tbody>
</table>
{{/each}}
{{/if}}
</div>
</body>
</html>
Output:
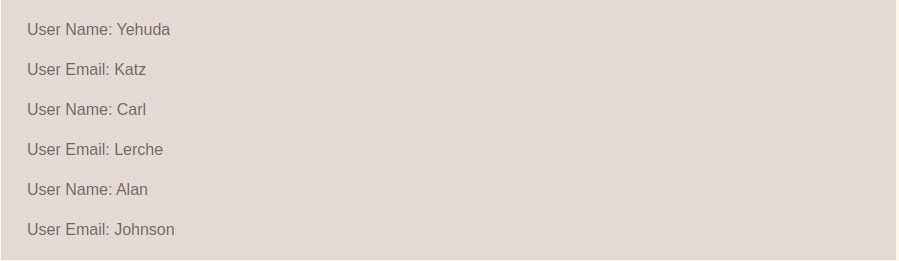
This is all well and good, but with this simple example maybe not so impressive. One of the other things that Handlebars includes are ‘helper’ expressions and one of these ‘helpers’ is the #each expression. This works just like a for Each loop in JavaScript. You add this to your template and then when you feed the template a series of Objects, the #each expression will iterate through each item and display information about it on the page.
Now in order to use helper you need to register them with handlebars.
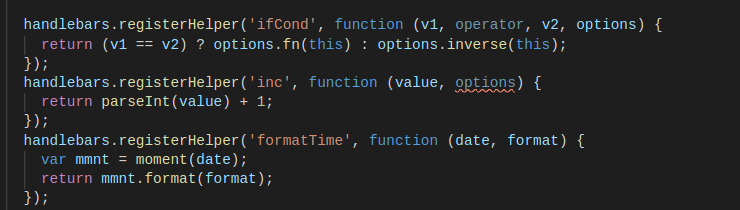
Now what if we want to render HTML in handlebars. nice question right?.
let's see how to render below HTML using handlebars.
JSON data:
{
title: "<p>Grade is the Characteristic Compressive strength of examination</p>"
}
handlebar template :
<div class="entry">
<h1>{{{title}}}</h1>
</div>
Output:

Conclusion
One of the great things about Handlebars is that it has tried to be 'bare-bones' as much as possible. While this may mean that it doesn't have all the features you may want or need, it is also flexible enough to add in those if you wish.
When you add in a helper be aware that you're effectively adding logic to a logic less templating system. You should evaluate whether it makes more sense to add the logic directly into your view instead, as a number of things can be performed there first. But this should be enough to get you started!