Dynamic Links using Firebase
Dynamic Links are like deep links into an app. it is work whether app is installed or not. When users open a Dynamic Link into an app that is not installed, then it is open either Play Store, App Store or any other web for install app,
After users install and open the app, the app displays the deep-linked content.
Dynamic Links mostly used in referral based sharing app, inviting other peoples or Sharing Certain Product. The benefit of mobile dynamic linking is the ability for marketers and app developers to bring users directly into the specific location within their app simple link.
Here We are Implement Dynamic Links using firebase so first basic step is to implement firebase into app
Project Setup
- First Thing We Need to Create Application on Firebase Console. Follow this link and create application.
- Add Firebase To React-Native
here we are, using react-native > 0.60 version and latest firebase version V6
to use dynamic links from firebase we also need firebase app dependency to install that follow below steps :
# Using npm
npm install --save @react-native-firebase/app
# Using Yarn
yarn add @react-native-firebase/app
After that install pod for ios for more information follow this link. just keep in mind that before you use any of the firebase service must install this module.
Above are pre required step to use dynamic links.
Install Dynamic Links
do run some command for install firebase dynamic link module like below :
# Install dynamic links module using npm
npm install @react-native-firebase/dynamic-links
Or
# Install dynamic links module using yarn
yarn add @react-native-firebase/dynamic-links
# For IOS app, run this command
cd ios/ && pod install
If you are using react native below 0.60 than do manual linking for android and ios both for that follow this link for IOS and for Android follow this link
When you are doing manual linking or get any androidX related issue than follow this link this can help.
Usage
usage is divided into two things
1) Create Dynamic Link
2) Handle Incoming Dynamic Link
Creating Dynamic Link :
Open the dynamic link tab from firebase console tab and click Get started
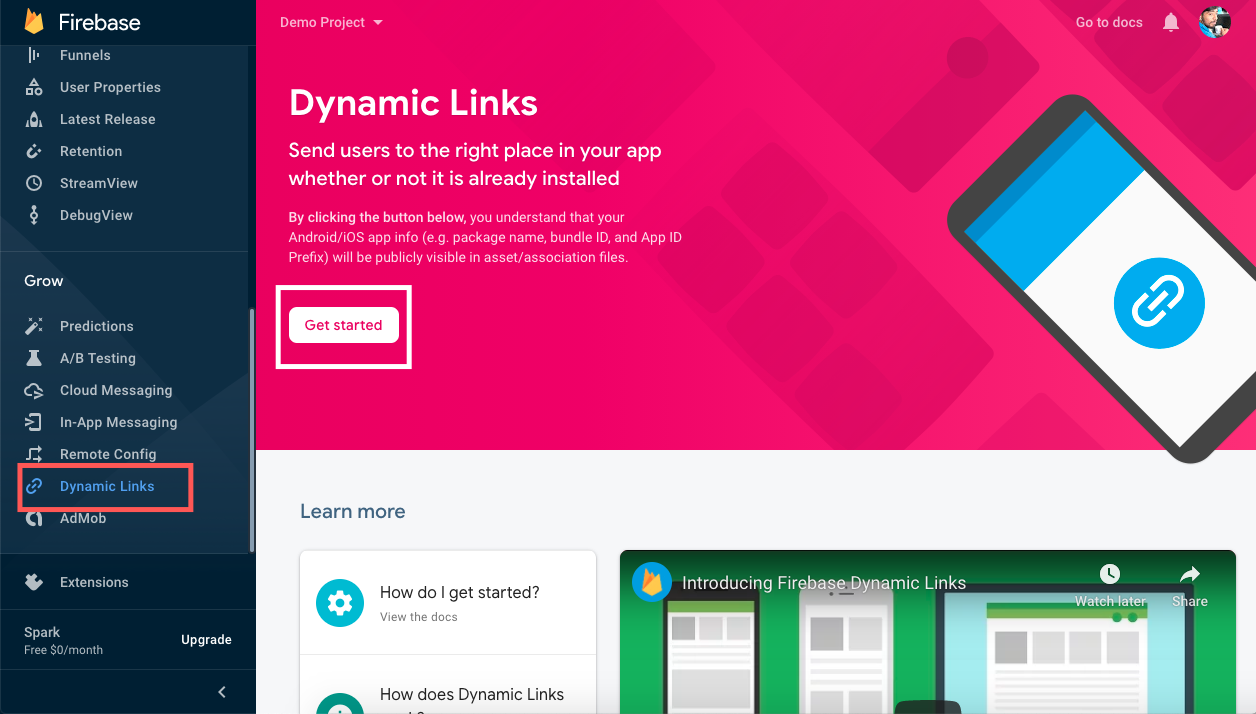
Add a domain. this is very important part let me explain in brief. there is two things first custom domain and second is firebase default provided domain. if you wants dynamic links on you website like my url is www.example.com in dynamic link than add that other wise firebase provide page.link domain like myDynamicLink.page.link which is actually not my website this domain is created by firebase. so than select link and continue.
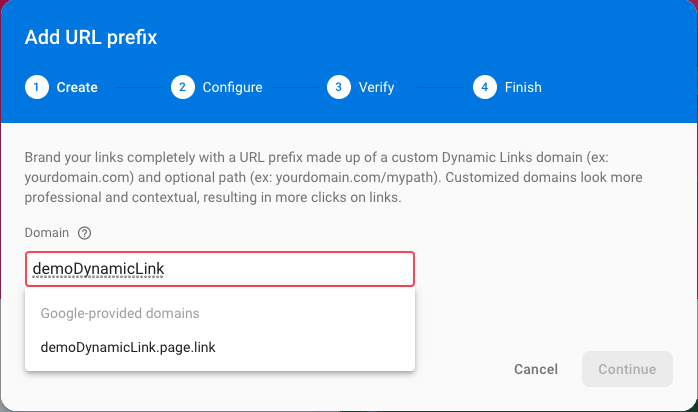
Now We can create Dynamic Links but before that we need to do one setup for IOS go to Project Settings => Select ios app and add App Store ID and Team ID
also add support email now we can good go.
- Check your domain is ready or not by using link [your domain]/apple-app-site-association in response you can see object with containing Apple Id.
- Goto Apple developer console enable Associate domain capabilities.
- For Custom domain also check this link
Click on New Dynamic Link and follow the steps. You can see Deep link url and Dynamic link name setup under setup dynamic link section. You can also pass params in link like in below image.
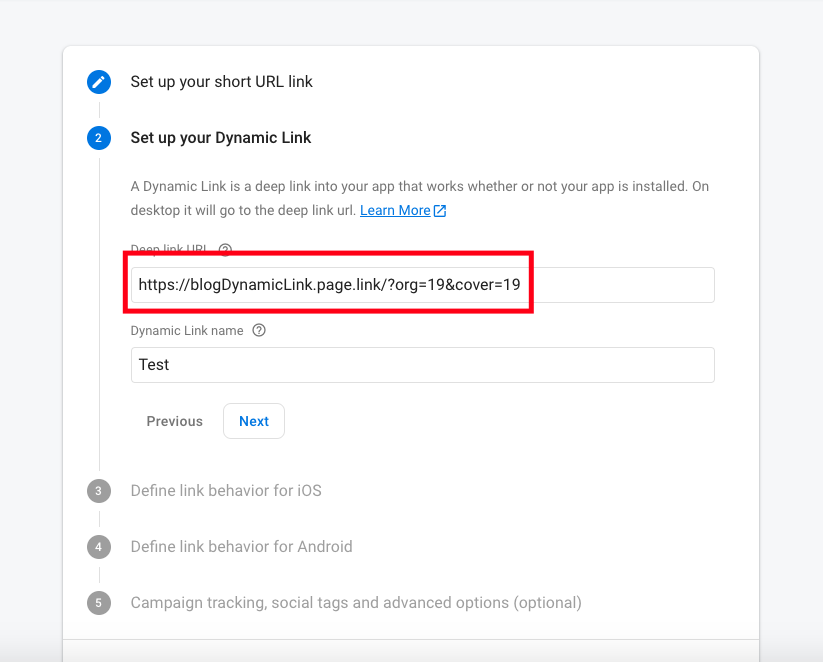
There is also some basic setup for dynamic link where do you open in app or browser, select according to your requirement.
We can Also Create Dynamic link Programmatically let's do that
# Install the dynamic-links module
import dynamicLinks from '@react-native-firebase/dynamic-links';
var link = dynamicLinks().buildShortLink(
{
link: 'https://blogdynamiclink.page.link/param=value',
domainUriPrefix: 'https://blogdynamiclink.page.link',
android: {
packageName: 'com.my.bundleId',
},
ios: {
appStoreId: '123456789',
bundleId: 'com.my.bundleId',
},
},
dynamicLinks.ShortLinkType.UNGUESSABLE,
);
its return dynamic link with shorter format. there are so much variation available you can check that on this link in create link section.
Handle Incoming Dynamic Link:
IOS Steps :
Open project in xcode and under TARGETS select Signing & capabilities tab check team and put associate domains in prefix with applinks:

Click the Info tab, and add Url Type to your project.
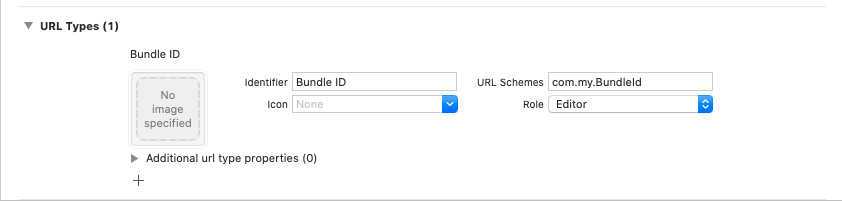
For Android :
Edit your AndroidManifest.xml file and update MainActivity tag.
<intent-filter>
<data android:scheme="https"
android:host="blogDynamicLink.page.link" />
</intent-filter>
Listening event in code:
- When app is in foreground
import dynamicLinks from '@react-native-firebase/dynamic-links';
componentDidMount() {
dynamicLinks().onLink((url) => {
// handle link inside app
});
}
- When app is in background or first time open
import dynamicLinks from '@react-native-firebase/dynamic-links';
componentDidMount() {
dynamicLinks().getInitialLink().then((link) => {
// handle link in app
});
}
Handling Errors
- double check AppId and TeamId firebase console.
- compare teamId in associate domains url [your domain]/apple-app-site-association
- also try remove app and restart mobile if not working.
Bonus
Firebase provide a way to verify the behaviour of dynamic links. add end of the firebase link add ?d=1 at the end and press enter it's opening chart of that link like below
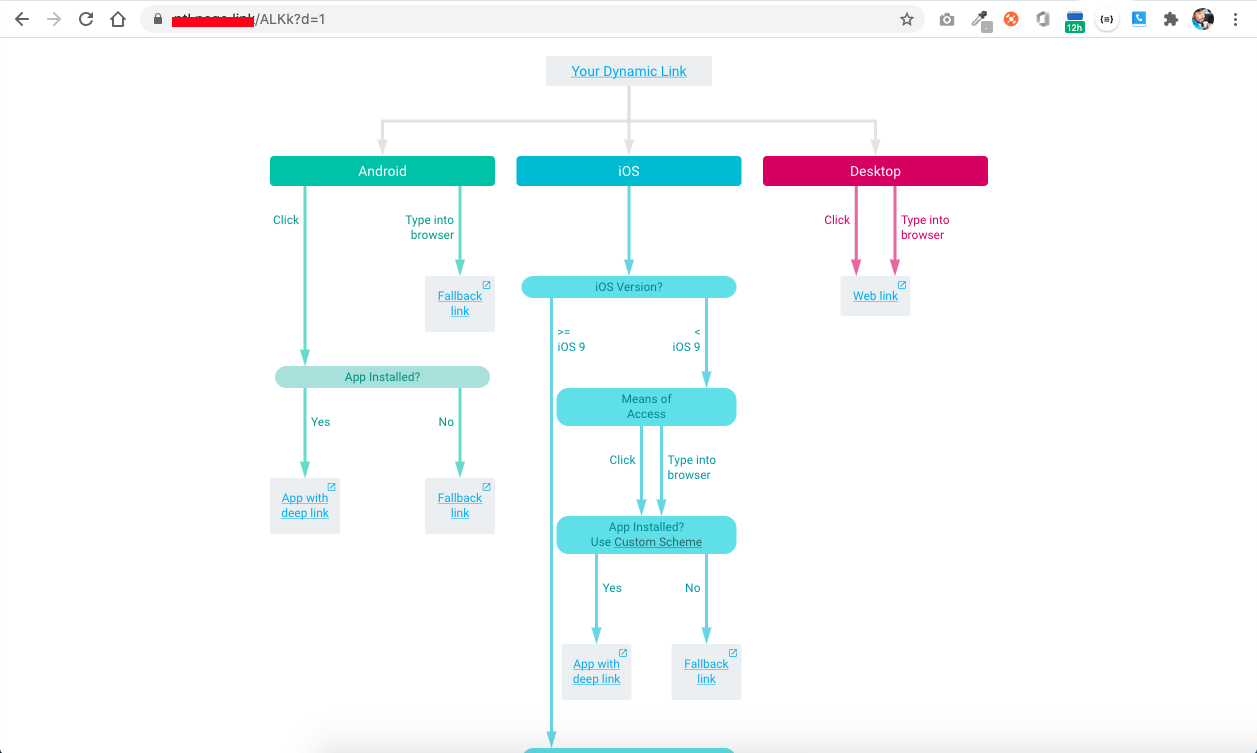