'@'mention integration with react native gifted chat
With this article we can learn about how to implement '@' mention in chat applications for mobiles with react-native-gifted-chat.
What is mention?
First question that comes to mind is that about mention, mention is mainly used for group chatting in that we can mention a member who's already join the group. We can also use it for giving suggestions of hashtags(for eg. #like, #friends)
when#
key triggered from keyboard it will showing a popup and we can select a suggested option from list of suggestions.
Firstly, initial process to start install react-native-gifted-chat using following:
- Using npm:
npm install react-native-gifted-chat --save
- Using Yarn:
yarn add react-native-gifted-chat
If you’re getting errors or want to get know more about props, methods and integration of this particular package please click on this
Now, after performing installation steps start implementation of react-native-gifted-chat
into your project as per your requirement.
Add gifted chat in your app
- By adding this into your app you can able to see chat input box and messages on your screen.
- In this all the props which are shown in code snippet are the basic props of gifted chat library.
- Add following code snippet into your file:
<GiftedChat messages={this.state.messages}
onSend={messages => this.onSend(messages)}
renderComposer={this.renderComposer}
listViewProps={{
style: styles.listContainer,
contentContainerStyle: styles.msgListContainer,
}}
user={{
_id: 1,
}}
renderMessage={(props) => {
return <MessageBubble messageObj={props.currentMessage}
position= {props.position} />}}
renderAvatar={null}
renderSend={this.renderSend}
text={this.state.messageText}
alwaysShowSend={true}
minComposerHeight={55}
maxComposerHeight={55}
bottomOffset={Platform.select({
ios: 200,
android: 0
})}
/>
- After adding above code snippet into your file it will create ui like below:
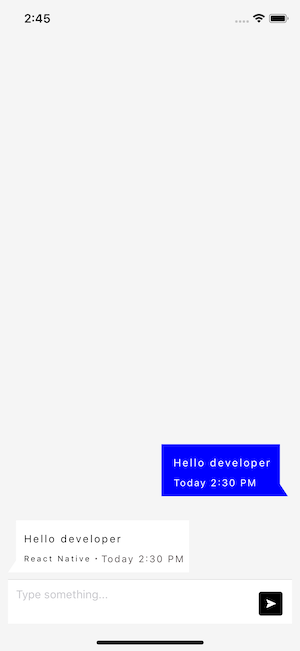
- For more UI styling and detail about screen please download project from link and refer
app.js
file
Integration of message composer
- For creating message composer import textInput component from react-native package and you can also use other input libraries for creating text-input. In this, I am using default text-input for message composer.
- From above code snippet of <GiftedChat/> please find renderComposer property. It looks like following:
renderComposer = (props) => {
return (
<View style={styles.composerContainer}>
<View style={styles.inputContainer}>
<TextInput {...props}
placeholder={'Type something...'}
ref={(input) => { this.msgInput = input; }}
onChangeText={(value) => this.onTextChange(value, props)}
style={styles.textInput}
value={props.text}
multiline={true}
/>
</View>
<Send {...props} containerStyle={styles.sendWrapperStyle} >
<View style={styles.sendContainer}>
<Image source={require('./images/Send.png')} style={styles.sendIconStyle} />
</View>
</Send>
</View>
)
}
- From above code onChangeText property will help to generate UI as following:
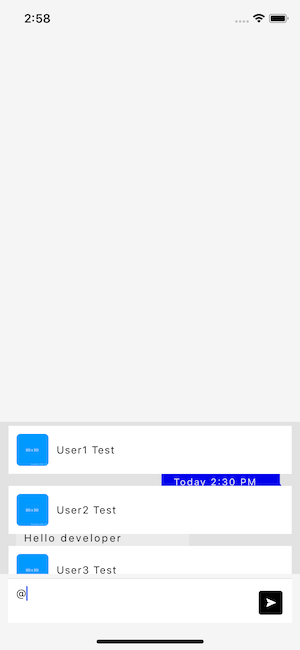
- onChangeText method containing following code:
onTextChange = (value, props) => {
const lastChar = this.state.messageText.substr(this.state.messageText.length - 1)
const currentChar = value.substr(value.length - 1)
const spaceCheck = /[^@A-Za-z_]/g
props.onTextChanged(value)
this.setState({
messageText: value
})
if(value.length === 0) {
this.setModalVisible(false)
} else {
if (spaceCheck.test(lastChar) && currentChar != '@') {
this.setModalVisible(false)
} else {
const checkSpecialChar = currentChar.match(/[^@A-Za-z_]/)
if (checkSpecialChar === null || currentChar === '@') {
const pattern = new RegExp(`\\B@[a-z0-9_-]+|\\B@`, `gi`);
const matches = value.match(pattern) || []
if (matches.length > 0) {
this.getUserSuggestions(matches[matches.length - 1])
this.setModalVisible(true)
} else {
this.setModalVisible(false)
}
} else if (checkSpecialChar != null) {
this.setModalVisible(false)
}
}
}
}
getUserSuggestions = (keyword) => {
this.setState({
isLoading: true
}, () => {
if(Array.isArray(userList)) {
if(keyword.slice(1) === '') {
this.setState({
userData: [...userList],
isLoading: false
})
} else {
const userDataList = userList.filter(obj => obj.name.indexOf(keyword.slice(1)) !== -1)
this.setState({
userData: [...userDataList],
isLoading: false
})
}
}
})
}
- By following above code, we can create suggestion list on trigger of specific keyword character
@
.
If you want to change triggering of @
you can replace all the @
inside onChangeText method and replace with your special characters like # + = $ % &
and it will trigger suggestion method whenever it will checks all the conditions.
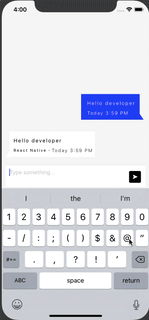
- By following above code it will help to integrate
@
mention with react-naitve-gifted-chat. - If you want code sample please download it from link. This will help you to understand key logic of
@
mention suggesting user name as a tappable list. - In this sample, I have also used for displaying suggestion panel react-native-modal package.
That's it. If you have any queries and want to ask any questions please ask me in comment section.
Thanks for reading my article. :)